Python 3 Continue Statement on Next Line
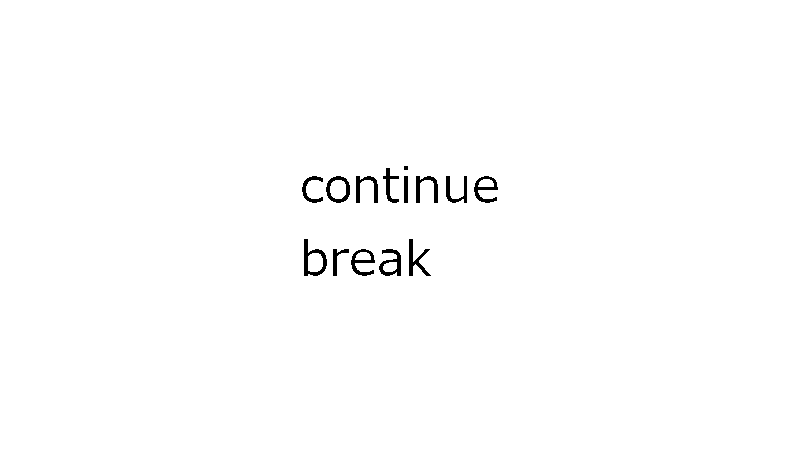
Hello everyone. This page is an English translation of a Japanese page. (The original Japanese has been slightly rewritten to make it easier to translate into English.)
This time we used Python's continue and break statements. These can be used in blocks of for and while statements (repetitive processing). For each of the continue and break statements, I made a sample program to check the behavior. This article is a reminder of what I learned from that experience.
The sample program uses for and if statements, about which I have a memorandum in articles "Python | Learned about for and while statements" and "Python | Learned the if statement (if, elif, else)".
Table of Contents
- continue statement
- break statement
- For a double loop
continue statement
The continue statement can be written in a block of for or while statements. When the continue statement is executed, the process moves to the next loop.
The following sample program is a simple program to check the operation of the continue statement.
Source
scores = [50, 52, 81, 69, 38] for score in scores: print('-----------------------------') print('Point...{0}'.format(score)) if score >= 80: continue print('Let's go for it.')
The execution result is as follows.
Execution results
----------------------------- Point...50 Let's go for it. ----------------------------- Point...52 Let's go for it. ----------------------------- Point...81 ----------------------------- Point...69 Let's go for it. ----------------------------- Point...38 Let's go for it.
In the above example, the variable "scores" stores the type "list". It is assumed that the list of scores is stored in this list type. The score is stored in the repeated variable score in the for statement. In the repetition process, if the score is less than 80, the specified message is output.
If the value of the score variable is 81, the continue statement will be executed. The process moves to the next loop. Therefore, the print statement written below the continue statement will not be executed.
The following is a table that shows whether the print statement was executed, for your reference.
The value of score | 50 | 52 | 81 | 69 | 38 |
---|---|---|---|---|---|
The first print statement | x | x | x | x | x |
The second print statement | x | x | x | x | x |
The third print statement | x | x | x | x |
The above sample program can also be written without using the continue statement. The same process can be done by reversing the condition of the if statement and writing a print statement directly in the if statement block.
Example of not using the continue statement
scores = [50, 52, 81, 69, 38] for score in scores: print('-----------------------------') print('Point...{0}'.format(score)) if score < 80: print('Let's go for it.')
However, the indentation of the print statement will be one step deeper.
If you are using an if statement to enclose the entire iteration process of a for statement, you may want to use a continue statement at the beginning to improve the outlook.
The following is a case where the iterative process of the for statement is two lines.
Source
scores = [50, 52, None, 69, 38] # When not using the continue statement for score in scores: if score is not None: print('Point') print(' ...{0}'.format(score)) print('----------------------------------------') # When using the continue statement for score in scores: if score is None: continue print('Point') print(' ...{0}'.format(score))
The execution result is as follows.
Execution results
Point ...50 Point ...52 Point ...69 Point ...38 ---------------------------------------- Point ...50 Point ...52 Point ...69 Point ...38
The above assumes that no processing will be done if the value of the score variable is None. In the second for statement, the indentation of the print statement is one step less.
break statement
When the break statement is executed in the for statement, the process exits the loop. For example, if the iterative process is just to find the desired value, you can avoid executing subsequent useless iterations.
The following sample program checks if there is a score of 80 or more in the list type variable "scores".
Source
scores = [50, 52, 81, 69, 38] score_first_passed = None for score in scores: print('Point...{0}'.format(score)) if score >= 80: score_first_passed = score break print('This score is a failing grade.') print('-----------------------------------') if score_first_passed is not None: print('The score for the first pass is {0}.'.format(score_first_passed)) else: print('There were no successful applicants.')
If the value of the variable score is 80 or more, the value is stored in the variable score_first_passed. After that, the break statement is executed and the iteration process ends there.
The execution result is as follows.
Execution results
Point...50 This score is a failing grade. Point...52 This score is a failing grade. Point...81 ----------------------------------- The score for the first pass is 81.
The reason why the first print statement in the for statement block is only printed up to the third time is because the break statement has been executed. Also, the print statement written below the break statement will not be executed.
The following is a table that shows whether the print statement was executed, for your reference.
The value of score | 50 | 52 | 81 | 69 | 38 |
---|---|---|---|---|---|
The first print statement | x | x | x | ||
The second print statement | x | x |
When the break statement is executed, the process exits the loop, so the second print statement is not executed in the process of score 81. Processing for scores 69 and 38 will also not be performed.
For a double loop
The continue statement skips the innermost loop, and the break statement exits the innermost loop.
The following is a sample program just to confirm the above.
Source
my_range = range(1, 6); for i in my_range: for j in my_range: print('{0:>3}'.format(i * j), end='') print() print('-----------------------------------') for i in my_range: for j in my_range: if i == 3 and j == 3: continue print('{0:>3}'.format(i * j), end='') print() print('-----------------------------------') for i in my_range: for j in my_range: if i == 3 and j == 3: break print('{0:>3}'.format(i * j), end='') print()
In the above for statement, variables i and j will be stored from 1 to 5. The first for statement outputs the multiplication table. In the second for statement, when the value of variable i is 3 and the value of variable j is 3, the continue statement is executed. The third for statement executes the break statement with the same condition.
The output results are as follows.
Execution results
1 2 3 4 5 2 4 6 8 10 3 6 9 12 15 4 8 12 16 20 5 10 15 20 25 ----------------------------------- 1 2 3 4 5 2 4 6 8 10 3 6 12 15 4 8 12 16 20 5 10 15 20 25 ----------------------------------- 1 2 3 4 5 2 4 6 8 10 3 6 4 8 12 16 20 5 10 15 20 25
In the second for statement, the value 9 is not printed because the continue statement is executed in the loop of the third stage output. Since the inner loop is skipped, the values 12 and 15 are output.
In the third for statement, the break statement is executed in the loop of the third stage output, so only up to the value 6 is output. This is because the process has left the inner loop.
That's all. I hope this is helpful to you.
Source: https://java-beginner.com/en/python-continue-break/
0 Response to "Python 3 Continue Statement on Next Line"
Post a Comment